本教程提供很多游戏使用的超大数值显示的解决方案
例如:
1 2 3 4 5 6 7 |
100=>100 1000=>1.00K 10000=>10.00K 100000=>100.00K 1000000=>1.00M 10000000=>10.00M 1000000000000=>1.00AA |
上代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 |
using System.Collections; using System.Collections.Generic; using System.Text; using UnityEngine; public static class NumberExtension { #region 转换大数值 private static string[] toLargeNumSign = new string[] {"K", "M", "B", "T", "AA", "AB", "AC", "AD", "AE", "AF", "AG", "AH", "AI", "AJ", "AK", "AL", "AM", "AN", "AO", "AP", "AQ", "AR", "AS", "AT", "AU", "AV", "AW", "AX", "AY", "AZ" , "BA", "BB", "BC", "BD", "BE", "BF", "BG", "BH", "BI", "BJ", "BK", "BL", "BM", "BN", "BO", "BP", "BQ", "BR", "BS", "BT", "BU", "BV", "BW", "BX", "BY", "BZ" , "CA", "CC", "CC", "CD", "CE", "CF", "CG", "CH", "CI", "CJ", "CK", "CL", "CM", "CN", "CO", "CP", "CQ", "CR", "CS", "CT", "CU", "CV", "CW", "CX", "CY", "CZ" , "DA", "DD", "DC", "DD", "DE", "DF", "DG", "DH", "DI", "DJ", "DK", "DL", "DM", "DN", "DO", "DP", "DQ", "DR", "DS", "DT", "DU", "DV", "DW", "DX", "DY", "DZ" }; public static string ToLargeNum(this int value) { if (value < 1000 && value > -1000) return value.ToString(); string str = value.ToString("#"); return GetToLargeNum(str); } public static string ToLargeNum(this long value) { if (value < 1000 && value > -1000) return value.ToString(); string str = value.ToString("#"); return GetToLargeNum(str); } public static string ToLargeNum(this float value) { if (value < 1000 && value > -1000) return value.ToString(); string str = value.ToString("#"); return GetToLargeNum(str); } public static string ToLargeNum(this double value) { if (value < 1000 && value > -1000) return value.ToString(); string str = value.ToString("#"); return GetToLargeNum(str); } private static string GetToLargeNum(string str) { bool isNegative = str[0] == '-'; if (isNegative) str = str.Remove(0, 1); int count = str.Length - 4; string sign = toLargeNumSign[Mathf.FloorToInt(count / 3f)]; int dotLeftCount = count % 3 + 1; StringBuilder valueStr = new StringBuilder(); if (dotLeftCount == 1) { valueStr.Append(str[0]); valueStr.Append('.'); valueStr.Append(str[1]); valueStr.Append(str[2]); } else if (dotLeftCount == 2) { valueStr.Append(str[0]); valueStr.Append(str[1]); valueStr.Append('.'); valueStr.Append(str[2]); valueStr.Append(str[3]); } else if (dotLeftCount == 3) { valueStr.Append(str[0]); valueStr.Append(str[1]); valueStr.Append(str[2]); valueStr.Append('.'); valueStr.Append(str[3]); valueStr.Append(str[4]); } valueStr.Append(sign); if (isNegative) return '-' + valueStr.ToString(); else return valueStr.ToString(); } #endregion } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Test : MonoBehaviour { // Start is called before the first frame update void Start() { Debug.Log(123 + " " + 123.ToLargeNum()); Debug.Log(1234 + " " + 1234.ToLargeNum()); Debug.Log(12345 + " " + 12345.ToLargeNum()); Debug.Log(123456 + " " + 123456.ToLargeNum()); Debug.Log(1234567 + " " + 1234567.ToLargeNum()); int i = int.MaxValue; Debug.Log("Int:" + i.ToString("#") + " " + i.ToLargeNum()); long l = long.MaxValue; Debug.Log("Long:" + l.ToString("#") + " " + l.ToLargeNum()); float f = float.MaxValue; Debug.Log("Float:" + f.ToString("#") + " " + f.ToLargeNum()); double d = double.MaxValue; Debug.Log("Double:" + d.ToString("#") + " " + d.ToLargeNum()); } } |
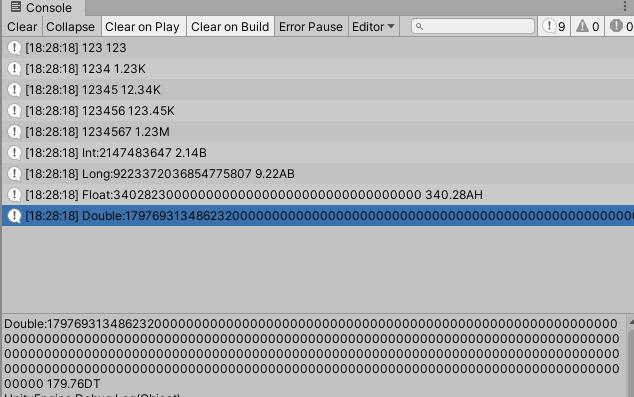
- 本文固定链接: http://www.u3d8.com/?p=2033
- 转载请注明: 网虫虫 在 u3d8.com 发表过