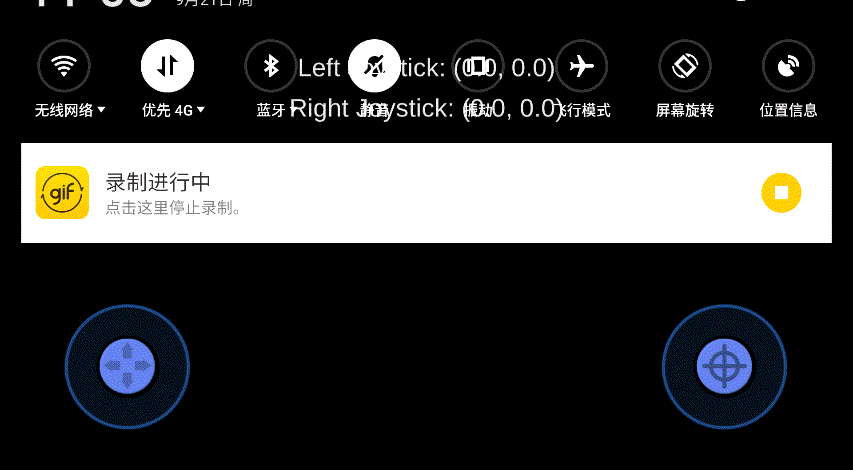
上代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
using UnityEngine; using UnityEngine.EventSystems; public class JoystickCtrl : MonoBehaviour, IDragHandler, IPointerUpHandler, IPointerDownHandler { /* component refs */ public RectTransform background; public RectTransform handle; /* public vars */ public float handleLimit = 1f; protected Vector2 inputVector = Vector2.zero; public float Horizontal { get { return inputVector.x; } } public float Vertical { get { return inputVector.y; } } public Vector2 Direction { get { return new Vector2(Horizontal, Vertical); } } public virtual void OnDrag(PointerEventData eventData) { } public virtual void OnPointerDown(PointerEventData eventData) { } public virtual void OnPointerUp(PointerEventData eventData) { } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.Events; using UnityEngine.EventSystems; public class JoystickComponentCtrl : JoystickCtrl { /* component refs */ public RectTransform Container; /* private vars */ private Vector2 _joystickCenter = Vector2.zero; private Vector3 _containerDefaultPosition; public UnityEvent OnTap; void Start() { this._containerDefaultPosition = this.Container.position; } public override void OnDrag(PointerEventData eventData) { Vector2 direction = eventData.position - _joystickCenter; inputVector = (direction.magnitude > background.sizeDelta.x / 2f) ? direction.normalized : direction / (background.sizeDelta.x / 2f); handle.anchoredPosition = (inputVector * background.sizeDelta.x / 2f) * handleLimit; } public override void OnPointerDown(PointerEventData eventData) { Container.position = eventData.position; handle.anchoredPosition = Vector2.zero; _joystickCenter = eventData.position; } public override void OnPointerUp(PointerEventData eventData) { Container.position = this._containerDefaultPosition; handle.anchoredPosition = Vector2.zero; inputVector = Vector2.zero; this.OnTap.Invoke(); } } |
GitHub下载地址:
https://github.com/654306663/TwoJoystickPro
- 本文固定链接: http://www.u3d8.com/?p=2088
- 转载请注明: 网虫虫 在 u3d8.com 发表过